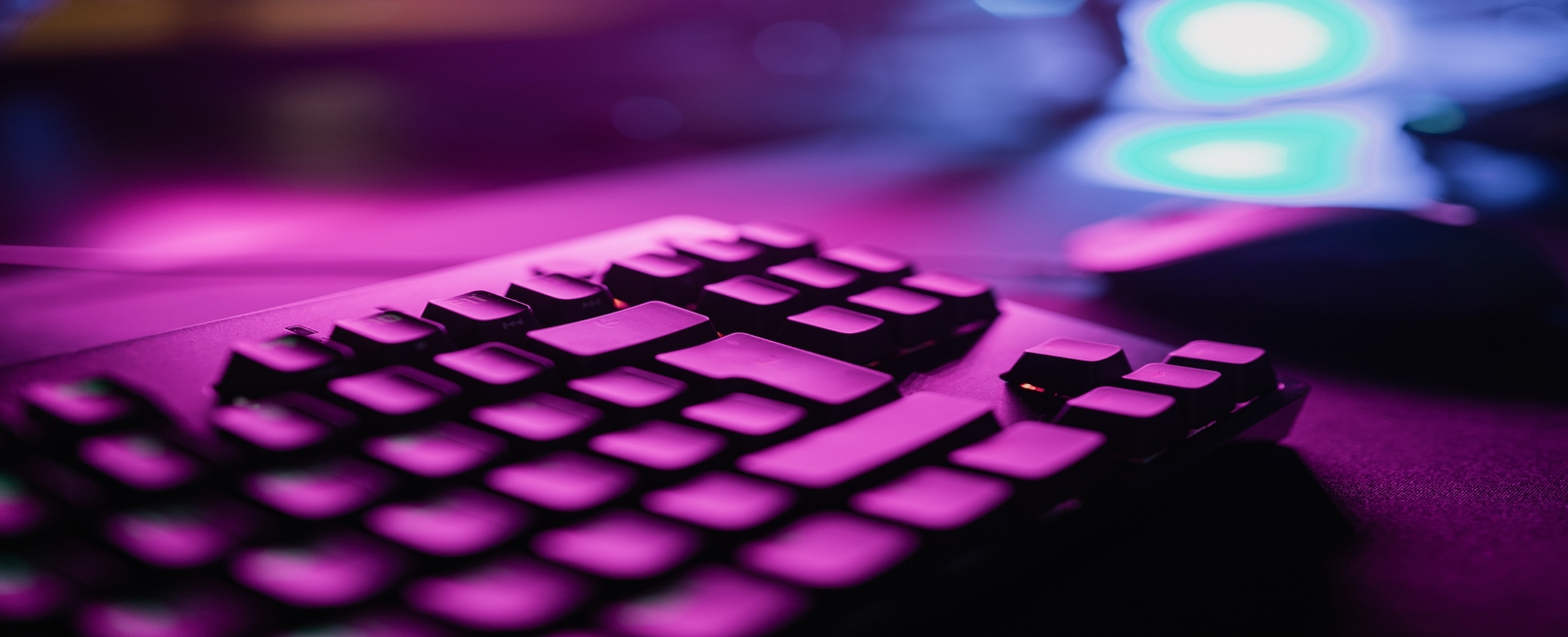
Do you want to automatically hide your keyboard whenever you touch outside it? Please continue reading and you will find out how to do it!
First, we will create our AutoHideKeyboardEditText class like the following:
public class AutoHideKeyboardEditText extends EditText {public AutoHideKeyboardEditText(Context context) {super(context);init();}public AutoHideKeyboardEditText(Context context, AttributeSet attrs) {super(context, attrs);init();}public AutoHideKeyboardEditText(Context context, AttributeSet attrs, int defStyleAttr) {super(context, attrs, defStyleAttr);init();}private void init() {setOnFocusChangeListener(new OnFocusChangeListener() {@Override public void onFocusChange(View v, boolean hasFocus) {if (!hasFocus) {hideKeyboard(v);}}});}private void hideKeyboard(View view) {InputMethodManager inputMethodManager = (InputMethodManager) view.getContext().getSystemService(Activity.INPUT_METHOD_SERVICE);inputMethodManager.hideSoftInputFromWindow(view.getWindowToken(), 0);}}
Whatever Constructor is used, we set an onFocusChangeListener to hide the keyboard using the proper method if we touch outside the EditText area (if the focus is not anymore on it).
Afterwards, create a layout similar to this
<LinearLayoutxmlns:android="http://schemas.android.com/apk/res/android"android:layout_width="match_parent"android:layout_height="match_parent"android:clickable="true"android:focusableInTouchMode="true"android:orientation="vertical"/>
being sure, whatever layout you want to use, to add clickable and focusableInTouchMode attributes with true value.
Now just add our AutoHideKeyboardTextView inside that layout. You can simply add it using XML like the following
<com.w3ma.androidshowcase.autohidekeyboard.AutoHideKeyboardEditTextandroid:layout_width="match_parent"android:layout_height="wrap_content"/>
You are done! Whenever you touch outside the EditText the keyboard will be automatically dismissed!
Please note: you should add the clickable and focusableInTouchMode attributes to the outermost view but if it is a ScrollView this might not work. For such case, those attributes could be added to the view directly under the ScrollView.
Full source code on my GitHub repository here.
I don’t know why this wasn’t voted as the best answer because it is indeed.
Quick Links